Mastering Animated Butterfly Charts with JavaScript
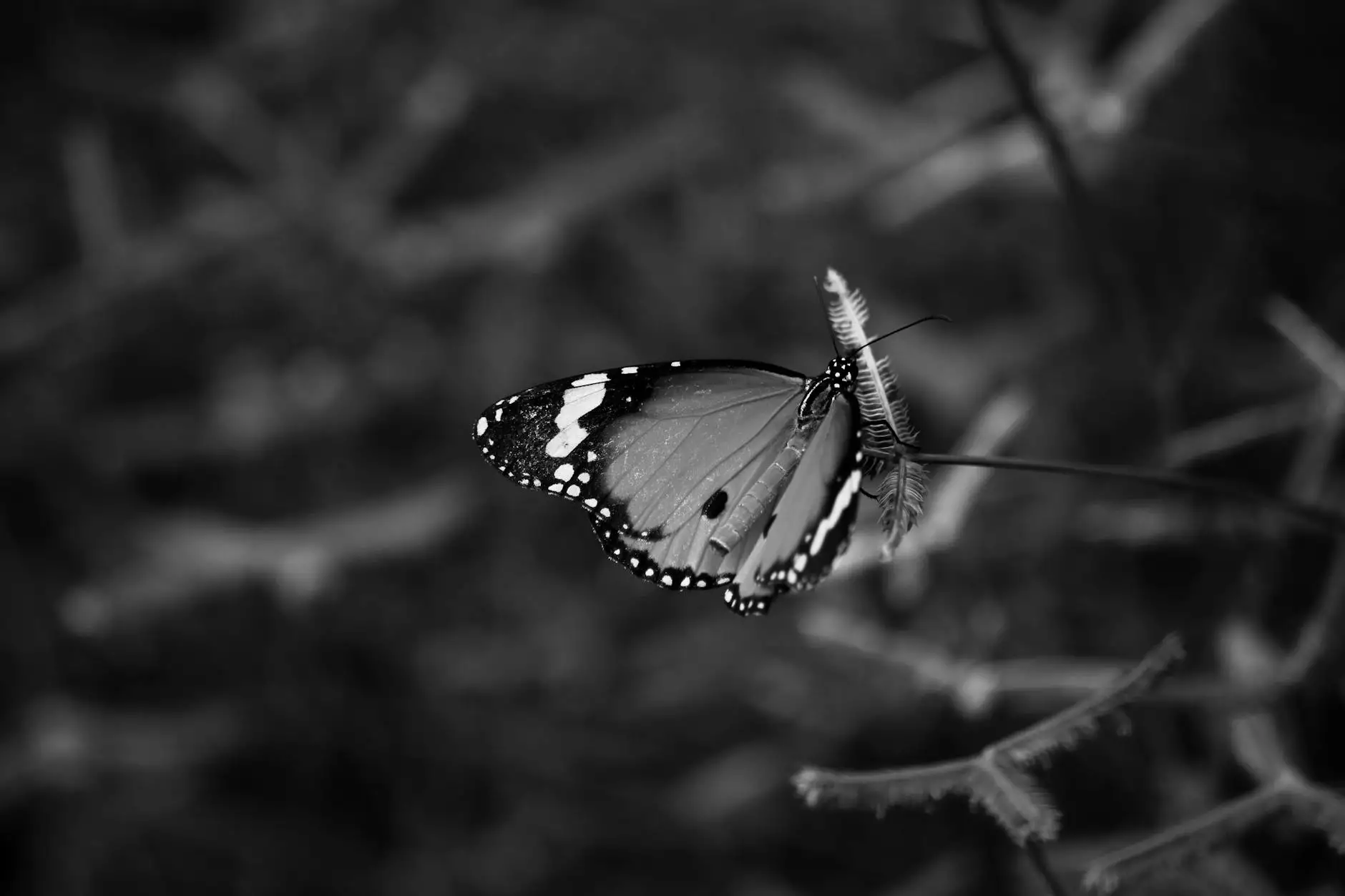
In the modern business landscape, visual data representation has become essential for effective communication and analysis. As such, animated butterfly charts represent a unique and powerful tool for visualizing comparative data, particularly in measuring two sides of a dataset. This article dives deep into the intricacies of creating and utilizing animated butterfly charts using JavaScript, while emphasizing their significance in business consulting and marketing strategies.
Understanding Butterfly Charts
A butterfly chart, also known as a back-to-back bar chart, is a specialized type of bar chart that allows users to compare two related sets of data side by side. It is particularly effective in showcasing demographic data, survey results, and any other information that can benefit from a comparative analysis.
Benefits of Using Butterfly Charts
- Visual Clarity: Provides a clear visual comparison of two datasets.
- Ease of Interpretation: Simplifies complex data into understandable visuals.
- Dynamic Insights: Allows for animated elements that engage viewers and highlight differences effectively.
Why Use Animated Butterfly Charts in Business?
Animations can bring a butterfly chart to life, allowing businesses to convey narratives through data. Incorporating animation into your butterfly charts can enhance user engagement and ensure that critical data points draw attention when they change or update in real-time.
Applications in Marketing and Business Consulting
From a marketing perspective, animated butterfly charts can be used to:
- Analyze Market Trends: Compare historical and current market trends effectively.
- Survey Feedback Analysis: Visually compare responses between different demographics.
- Sales Data Visualization: Illustrate sales performance across two product categories or campaigns.
In business consulting, these charts serve as powerful decision-making tools, assisting consultants in:
- Risk Assessment: Assess potential risks versus rewards by visualizing data side by side.
- Performance Assessment: Compare organizational performance against competitors.
- Identifying Opportunities: Highlight gaps and areas for growth through visual data.
Creating Animated Butterfly Charts Using JavaScript
Creating an animated butterfly chart using JavaScript can be seamlessly executed with libraries such as Chart.js or D3.js. Let’s delve into a step-by-step guide to building this powerful visualization.
Step 1: Set Up Your Environment
To begin, ensure you have a basic HTML structure. Include references for the JavaScript libraries you will use.
```html Your Animated Butterfly Chart // Your JavaScript code will go here ```Step 2: Prepare Your Data
Before you start coding, prepare the data that will populate your charts. In this example, let's say we have two datasets representing males and females in various age groups. Represent your data in arrays:
```javascript const ageGroups = ['0-10', '11-20', '21-30', '31-40', '41-50', '51-60', '61+']; const maleData = [50, 60, 70, 80, 90, 100, 110]; const femaleData = [40, 55, 65, 75, 85, 95, 105]; ```Step 3: Configure the Chart
Now it’s time to configure the chart settings:
```javascript const ctx = document.getElementById('butterflyChart').getContext('2d'); const butterflyChart = new Chart(ctx, { type: 'bar', data: { labels: ageGroups, datasets: [{ label: 'Male', data: maleData, backgroundColor: 'rgba(54, 162, 235, 0.6)', borderColor: 'rgba(54, 162, 235, 1)', borderWidth: 1, // to create the butterfly effect, we set a negative value for the left side datalabels: { anchor: 'end', align: 'start' } }, { label: 'Female', data: femaleData.map(value => -value), // Negate values for the left side backgroundColor: 'rgba(255, 99, 132, 0.6)', borderColor: 'rgba(255, 99, 132, 1)', borderWidth: 1, datalabels: { anchor: 'end', align: 'start' } }] }, options: { scales: { xAxes: [{ stacked: true, ticks: { beginAtZero: true } }], yAxes: [{ stacked: true, ticks: { callback: function(value) { return Math.abs(value); // Show absolute values } } }] }, animation: { duration: 1000, // Animation duration easing: 'easeInOutQuad' // Animation easing } } }); ```Step 4: Add Animation & Interactivity
To enhance the storytelling aspect of your chart, you may want to add some interactivity or further animations. For example, you can create an event listener that displays detailed information when hovering over the bars:
```javascript options: { tooltips: { callbacks: { label: function(tooltipItems) { return tooltipItems.dataset.label + ': ' + Math.abs(tooltipItems.yLabel); } } } } ```Step 5: Testing and Optimization
Once implemented, test the chart in various browsers and screen sizes to ensure that it works correctly on all platforms. Optimize the performance by minimizing the dataset size and simplifying the animations if necessary.
Conclusion
In summary, animated butterfly charts built with JavaScript are invaluable tools for data visualization in business consulting and marketing applications. They allow businesses to engage viewers with dynamic and interactive presentations of data, facilitating better decision-making and deeper insights. As companies increasingly rely on data to drive strategies, mastering techniques like these will empower them to communicate effectively and capitalize on the rich narratives that data provides.
Investing time in learning tools such as Chart.js or D3.js will not only enhance your visual data representation skills but also position you as a leader in business analytics. Embrace the power of animated butterfly charts and watch your marketing and consulting capabilities soar.
animated butterfly chart js